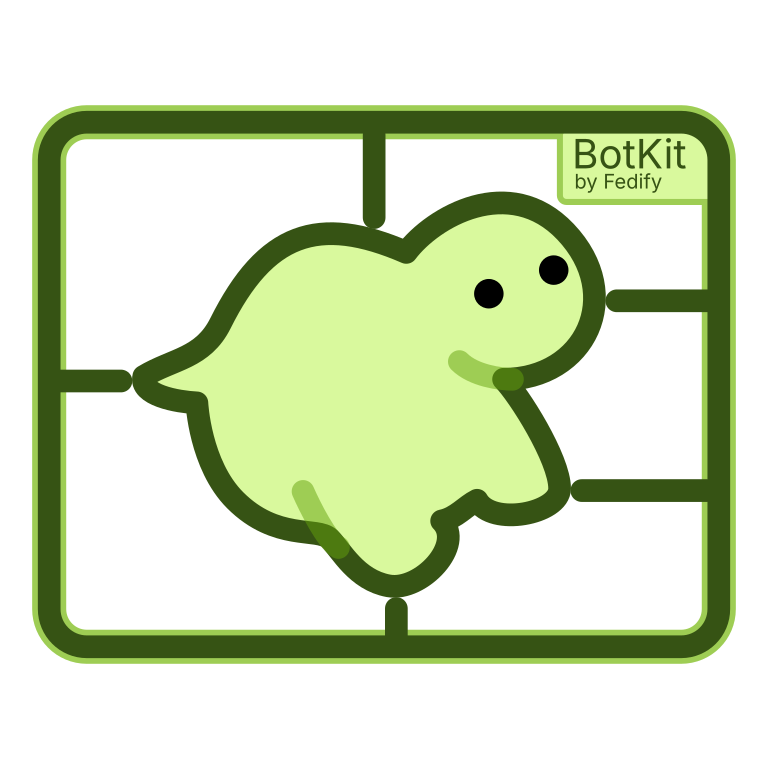
BotKit by Fedify 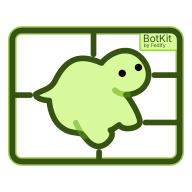
@botkit@hollo.social
We're excited to introduce emoji reactions in the upcoming #BotKit 0.2.0 release!
With the new Message.react()
method, your bot can now react to messages using standard Unicode #emojis:
await message.react(emoji`👍`);
#Custom_emoji support is also included, allowing your bot to react with server-specific emojis:
const emojis = bot.addCustomEmojis({
// Use a remote image URL:
yesBlob: {
url: "https://cdn3.emoji.gg/emojis/68238-yesblob.png",
mediaType: "image/png",
},
// Use a local image file:
noBlob: {
file: `${import.meta.dirname}/emojis/no_blob.png`,
mediaType: "image/webp",
},
});
await message.react(emojis.yesBlob);
Reactions can be removed using the AuthorizedReaction.unreact()
method:
const reaction = await message.react(emoji`❤️`);
await reaction.unreact();
Want to try these features now? You can install the development version from JSR today:
deno add jsr:@fedify/botkit@0.2.0-dev.84+c997c6a6
We're looking forward to seeing how your bots express themselves with this new feature!